Overview
PTMan is a desktop human resource application used for managing part-time employees.
PTMan aims to give part-time employees the freedom of choosing when they want to work by registering for the available shifts set by the manager.
Thereby reducing the hassle of work scheduling for both employers and employees.
The user interacts with it using a CLI, and it has a GUI created with JavaFX. It is written in Java, and has about 10 kLoC.
Summary of contributions
-
Major enhancement: Added access control that manages functions for employer and employee. [Issue #39]
-
What it does:
-
Assigns password to each employer and employee.
-
Separates command for employer and employee.
-
Implements API for password checking that needs password.
-
Implements Login/Logout to admin mode for employer to use admin commands at ease.
-
Implements Change/reset password for both group of user with email service.
-
-
Justification: This ensure only authenticated personnel can use the system and restrict employee to use functions that are meant for the employer. This prevent misuse of PTMan.
-
Highlights: This enhancement puts security in high regard. Lots of consideration is put into avoiding inconvience for the user while maintaining high security.
-
-
Minor enhancement: Added salary parameter and addsalary command for employer to manage employees' pay.
-
Code contributed: [Functional code] [Test code]
-
Other contributions:
-
Project management:
-
Constantly collate updates from the team and call for meeting if there is a need to.
-
-
Enhancements to existing features:
-
Community:
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Logging in to admin mode: login
Logs in to admin mode, allowing you to use all the manager commands.
Format: login pw/ADMIN_PASSWORD
Please remember to logout after you are done making changes.
This is to prevent unauthorised access to manager commands. Refer to Logging out of admin mode: logout for more information.
|
Guided Example:
Logging out of admin mode: logout
Logs out of admin mode, preventing further usage of manager commands.
Format: logout
Guided Example:
Changing the admin password: changeadminpw
Changes the password to enter admin mode.
Format: changeadminpw pw/CURRENT_PASSWORD pw/NEW_PASSWORD pw/CONFIRM_NEW_PASSWORD
Shorthand: cap
pw/CURRENT_PASSWORD pw/NEW_PASSWORD pw/CONFIRM_NEW_PASSWORD
To prevent unauthorised access to admin mode, managers should change the admin password when running PTMan for the first time. |
The password should be at least 8 characters long. You must key in the current and new password in the correct sequence as given in the format. |
Guided Example:
-
To change the password from
DEFAULT1
tohunter22
, type:changeadminpw pw/DEFAULT1 pw/hunter22 pw/hunter22
. The passwords will be masked by asterisks as shown in Figure 5.Figure 5. PTMan Admin Password Change Command -
After the password is successfully changed, a confirmation message will be displayed in the result display as seen in Figure 6.
Figure 6. Successful PTMan Admin Password Change
Resetting the admin password: resetadminpw
Resets the password to enter admin mode and sends a randomly generated password to the outlet’s email address.
You may use the new password to login and change to your preferred password.
Format: resetadminpw
Shorthand: rap
The randomly generated password will be sent to the outlet’s email address stored in PTMan. You can view this email address under the outlet display of the GUI. |
Guided Example:
Adding salary to an employee: addsalary
Adds a specified amount to an employee’s salary.
Format: addsalary EMPLOYEE_INDEX s/AMOUNT_TO_ADD
Shorthand: adds EMPLOYEE_INDEX s/AMOUNT_TO_ADD
Guided Example:
-
To increase the first employee’s salary by $100, key in the command as shown below in Figure 9.
Figure 9. PTMan Add Employee Salary Command -
Upon successful execution of the command, you will see a confirmation message in the result display and the applied salary as shown in Figure 10.
Figure 10. Successful Addition of Salary for Employee in PTMan
Employee’s salary cannot be deducted using this command. |
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Password Feature
Reason for implementation
As PTMan is currently designed to use locally on a system, both employer and employee must go through the same system to allocate their preferred slot or to edit the data in PTMan. A Password class is given to both employer and employee to ensure that they are the authorized person that is using the system.
How it is implemented
A Password class is created with two constructors.
new Password(); new Password(String hashCode);
new Password()
produce hash code converted by SHA-256 using the default password "DEFAULT1" and store it within the class.
new Password(String hashCode)
allows storage to directly insert the generated hash code to create the password class upon loading.
To create a password with desired password String, one can invoke the method below. |
createPassword(String password)
As of version 1.2, each person and outlet now has a Password
class. You may refer to Figure 11 for an overview of the Password
class.
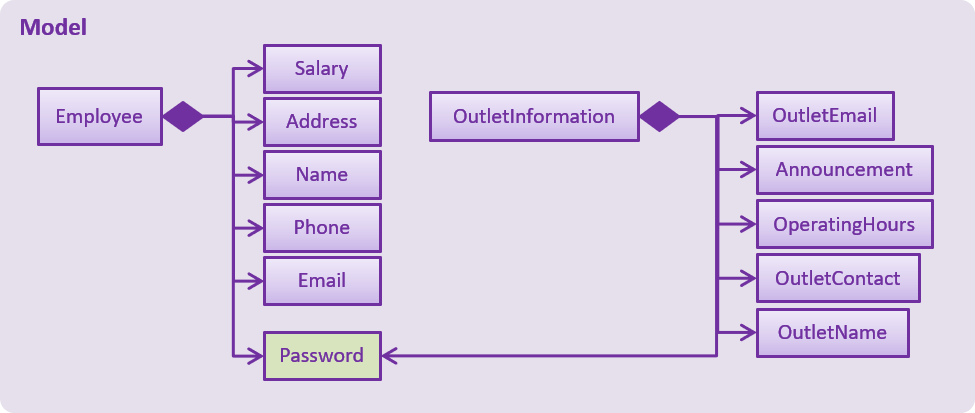
The reason of converting password string to hashCode is to protect user’s password from being seen in the storage file. Anyone who get hold of the data are not able to convert the hashCode back to the original password string as SHA-256 is a collision resistant hash.
Admin Mode and Command Feature
Reason for implementation
Initial startup of PTMan require huge amount of manual input by admin. To promote hassle-free commands, employers can log in to admin mode with login
command and use the command provided without the need to be autheticated for every command.
How it is implemented (admin mode)
Model component now implement three new API for logic component to use.
boolean isAdminMode(); boolean setTrueAdminMode(Password password); /** guarantee to set adminMode to false */ setFalseAdminMode();
setTrueAdminMode(Password password)
requires a password that will check against the outlet password and set admin mode accordingly. Failing to give the correct password will result in returning false.
How it is implemented (admin command)
In order to enable commands to be usable only in admin mode the code below must be added to the execution() of the command first.
if (!model.isAdminMode()) { throw new CommandException(MESSAGE_ACCESS_DENIED); }
Figure 12 below illustrates how the admin command is generally executed.
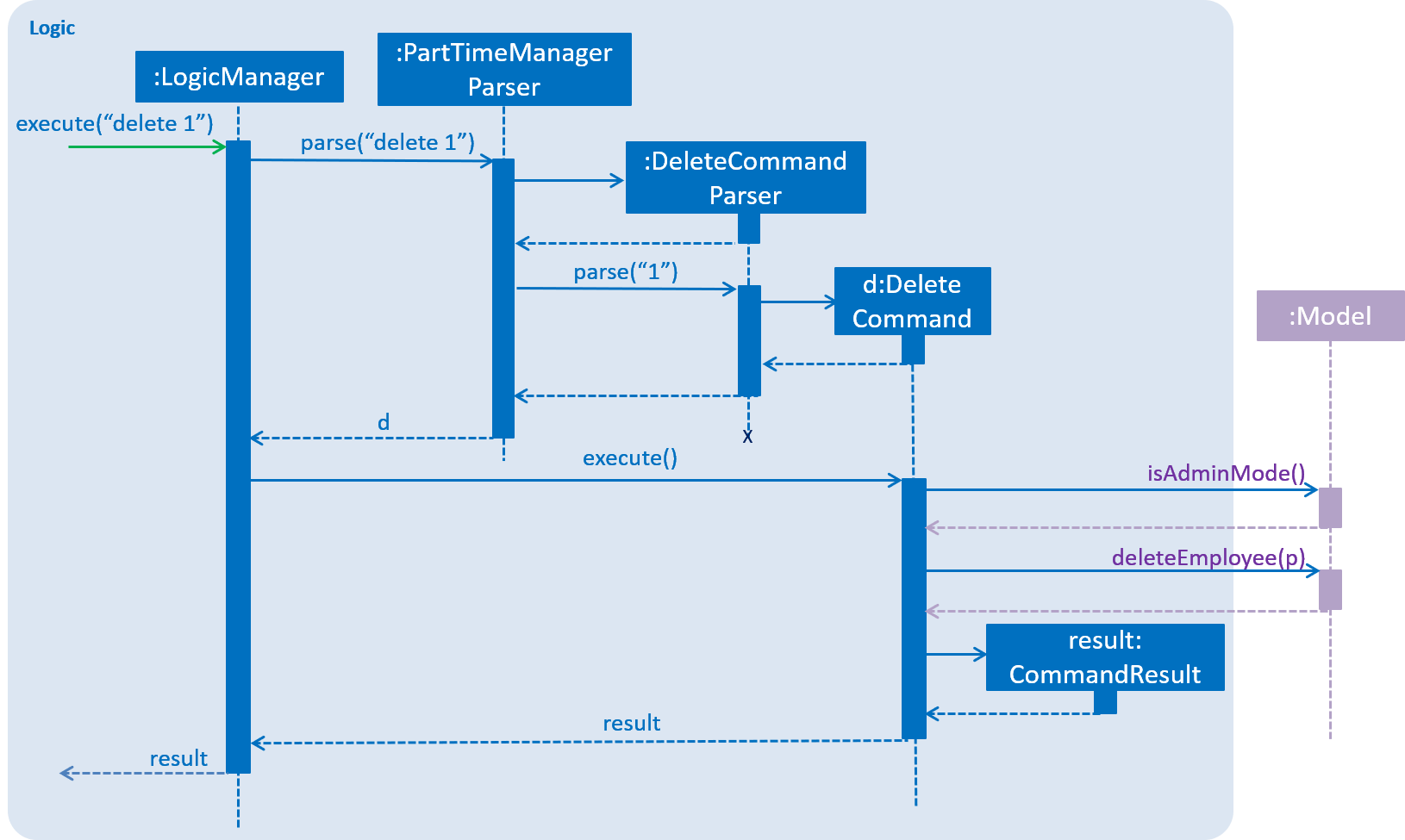
delete 1
Admin CommandEmail Service Feature
Reason for implementation
Email service can be useful to send reset password and notification to employee. To facilitate easy sending of email, a singleton EmailService
class is introduced.
How it is implementation
To get an instance of the email service the following code can be called anywhere.
EmailService.getInstance();
Currently, there are two specialized methods in EmailService
class that can be used to send email.
sendTimetableAttachment(String email, String filename) sendResetPasswordMessage(String name, String email, String newPassword)
sendTimetableAttachment
crafts a specialized email and send to the specified email
with attachment from filename
.
sendResetPasswordMessage
crafts a specialized email and send to the specified email
to the intended personnel.
EmailService class is free for extension.
|