Overview
PTMan is a desktop human resource application used for managing part-time employees.
PTMan aims to give part-time employees the freedom of choosing when they want to work by registering for the available shifts set by the manager.
Thereby reducing the hassle of work scheduling for both employers and employees.
The user interacts with the application using a CLI, and it has a GUI created with JavaFX. It is written in Java, and has about 10 kLoC.
Summary of contributions
-
Major enhancement: Added the shift feature.
-
What it does: Allows employers to create work shifts. Employees can then apply for the shifts that they want to work in.
-
Justification: This feature allows for easy management of manpower while giving employees the freedom and flexibility to work whenever they want to.
-
Highlights: This enhancement modifies the app’s model to store shifts. The storage component also had to be modified to save shifts to local storage. There were design considerations to integrate shifts into the existing model while minimizing coupling with the other components. To enable user interaction with this feature, various commands that manipulate shifts had to be implemented.
-
-
Minor enhancement: Added command aliases that are shorthands for the full commands.
-
Code contributed: [Functional code] [Test code]
-
Other contributions:
-
Project management:
-
Managed milestones and issue-tracking on GitHub.
-
-
Documentation:
-
Tools:
-
Integrated TravisCI to the team repo.
-
Integrated Coveralls to the team repo.
-
Integrated Codacy to the team repo.
-
-
Contributions to the User Guide
Given below are sections I contributed to the User Guide. They showcase my ability to write documentation targeting end-users. |
Adding a shift: addshift
Adds a shift to the timetable to indicate that you require employees at that period.
Format: addshift d/DATE ts/START_TIME te/END_TIME c/EMPLOYEE_CAPACITY
Shorthand: as d/DATE ts/START_TIME te/END_TIME c/EMPLOYEE_CAPACITY
Guided Example:
-
To add a shift on 13th April 2018 from 12pm to 6pm that requires 3 employees, execute the command
addshift d/13-04-18 ts/1200 te/1800 c/3
as shown in Figure 1.Figure 1. PTMan Add Shift Command -
You should see a confirmation message in the result display along with the shift you’ve added being displayed in the timetable. This is illustrated in Figure 2.
Figure 2. Successful Adding of a Shift in PTMan
Deleting a shift: deleteshift
Deletes a shift from the timetable.
Format: deleteshift SHIFT_INDEX
Shorthand: ds SHIFT_INDEX
Guided Example:
-
If you wish to delete shift 1, execute the command
deleteshift 1
as shown in Figure 3 below.Figure 3. PTMan Delete Shift 1 Command -
Upon successful deletion, you will see a confirmation message in the result display and you can no longer see the shift in the timetable. Figure 4 illustrates the successful deletion of shift 1.
Figure 4. Successful Deletion of Shift 1 in PTMan
Contributions to the Developer Guide
Given below are sections I contributed to the Developer Guide. They showcase my ability to write technical documentation and the technical depth of my contributions to the project. |
Shifts Feature
Reason for implementation
PTMan is designed to give employees the freedom and flexibility to choose the shifts they want to work in. By allowing employers to add or delete shifts, employees can then apply for the shifts that are available.
How it is implemented
The Shift
class represents a shift in PTMan.
It stores:
-
The
Date
of the shift.Date
is a class that wraps Java’sLocalDate
class. -
The starting and ending
Time
of the shift.Time
is a class that wraps Java’sLocalTime
class. -
The employee
Capacity
for the shift.Capacity
is a class that wraps an integer. -
The
UniqueEmployeeList
of employees working in the shift.UniqueEmployeeList
is a list that stores theEmployee
objects of employees that have applied for the shift. It guarantees there are no duplicate employees in the shift.
Figure 5 is a class diagram that displays the association between Shift
and other components in the Model
.
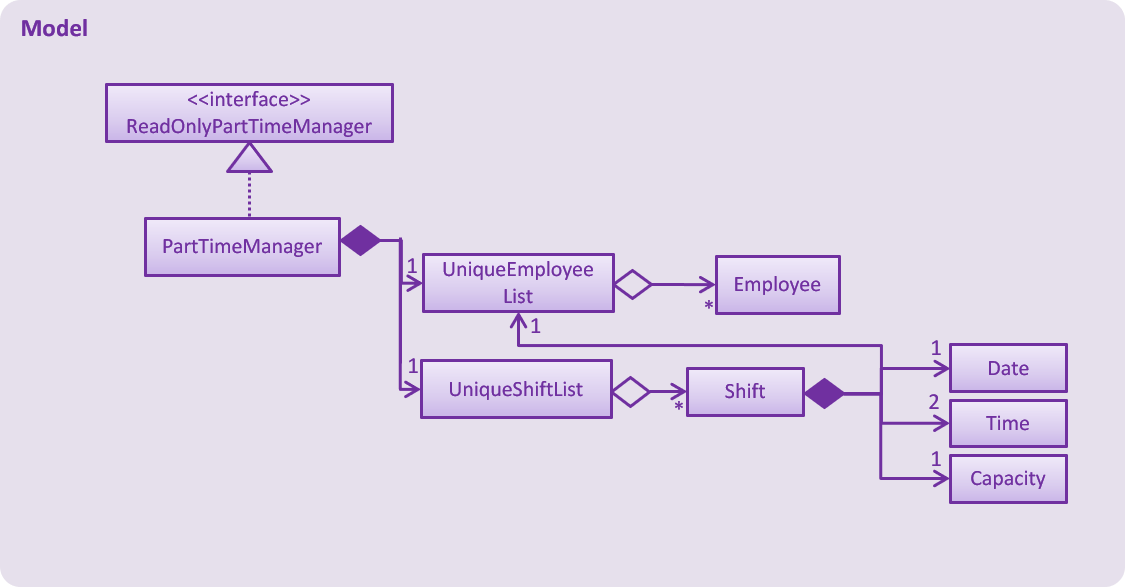
Date
and Time
use Java’s LocalDate
and LocalTime
classes for easy integration with the timetable.
They also make formatting and parsing simple through the use of Java’s DateTimeFormatter
.
To store a list of shifts in PartTimeManager
, we use a UniqueShiftList
to ensure there are no duplicate shifts.
Commands
The following are commands that directly interact with the Shift
class:
-
AddShiftCommand
: Creates aShift
and adds it to theUniqueShiftList
inPartTimeManager
. -
DeleteShiftCommand
: Deletes aShift
from theUniqueShiftList
inPartTimeManager
. -
ApplyCommand
: Adds anEmployee
to theUniqueEmployeeList
in theShift
.
To adhere to defensive programming practices, instead of simply adding theEmployee
to theShift
,ApplyCommand
does the following:-
Create a copy of the specified
Shift
-
Add the
Employee
to the copy. -
Replace the original
Shift
with the copy.
-
-
UnapplyCommand
: Removes anEmployee
from theUniqueEmployeeList
in theShift
.
Similar toApplyCommand
,UnapplyCommand
will:-
Create a copy of the specified
Shift
. -
Remove the
Employee
from the copy. -
Replace the original with the copy.
-
Shift indexing
The commands DeleteShiftCommand
, ApplyCommand
and UnapplyCommand
access the specified Shift
via it’s index displayed on the timetable.
The preferred behaviour for the indexes is to have the first shift of the week start from index 1, with subsequent shifts incrementing that index.
However, having shifts that are earlier than the current timetable week will cause the first shift of the week to have an index that is greater than 1.
As seen in Figure 6 below, the shift on Monday has index 5 because there are 4 other shifts in the week(s) before the current week.
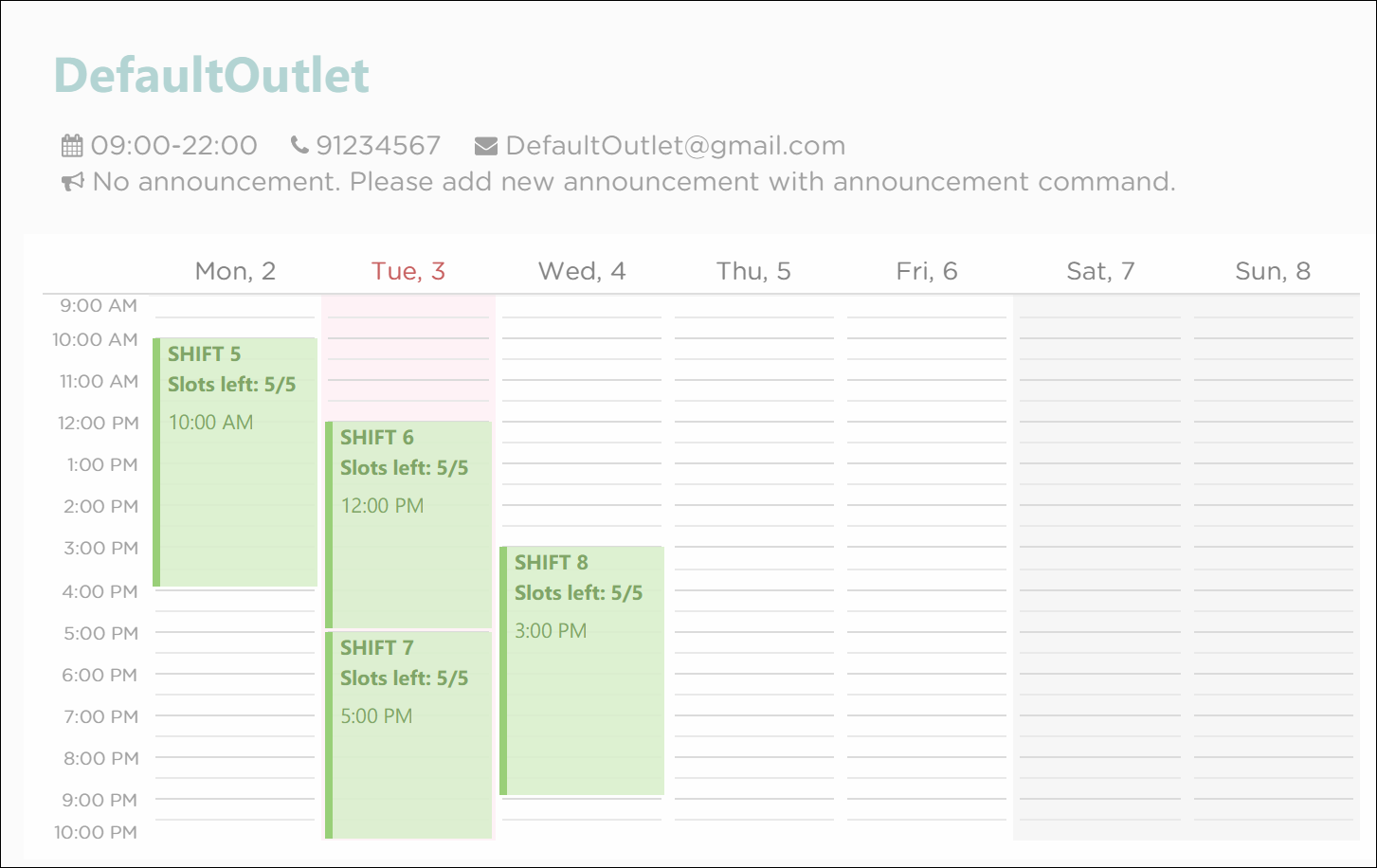
To avoid this, we only want to index shifts that are visible in the current timetable view.
This can be achieved by setting the Predicate<Shift>
for the FilteredList<Shift>
in ModelManager
to filter shifts in the current week as shown below:
// In Model public static Predicate<Shift> PREDICATE_SHOW_WEEK_SHIFTS = shift -> getWeekFromDate(shift.getDate().getLocalDate()) == getWeekFromDate(LocalDate.now()); // In ModelManager updateFilteredShiftList(PREDICATE_SHOW_WEEK_SHIFTS);
This results in the desired shift indexing as shown in Figure 7 below.
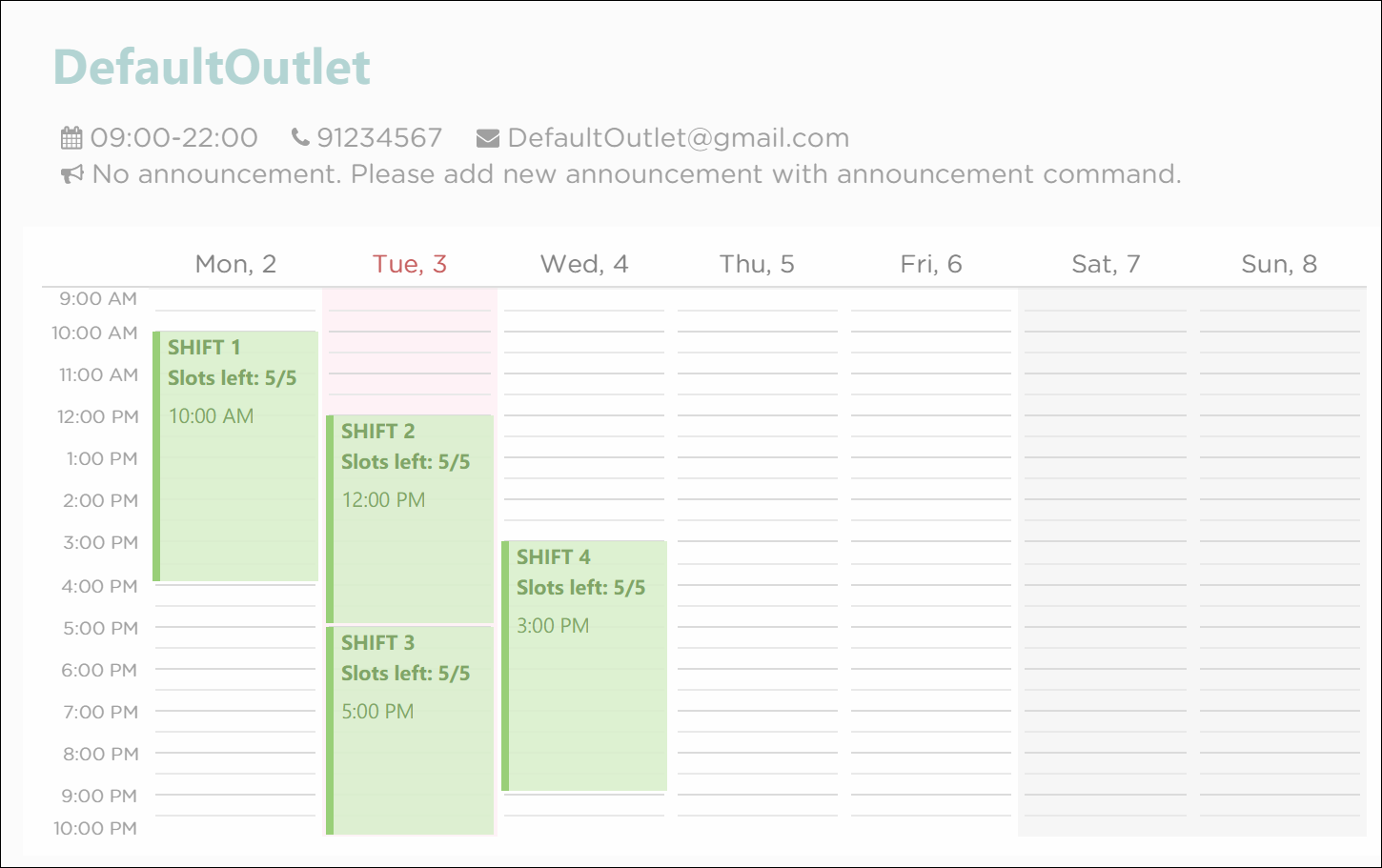